Table Of Content
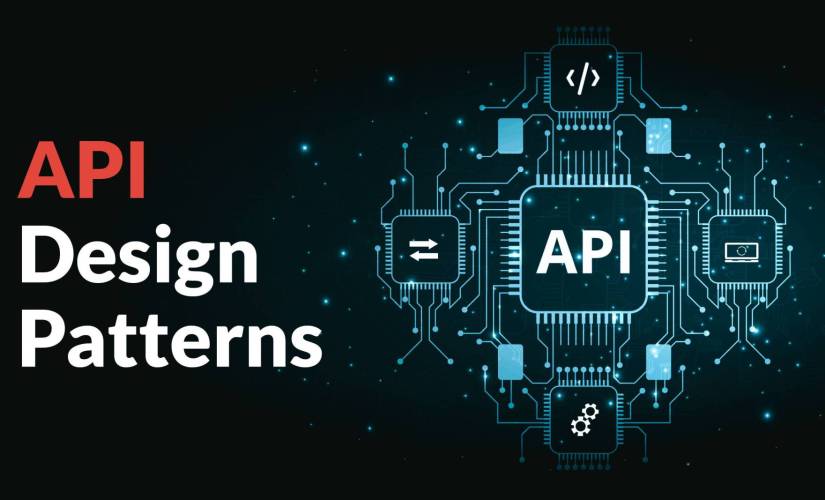
When the same request is made again, the client can return the cached response instead of making the request again. Conversely, server-side caching, the server stores the response and makes it available when the request is made again instead of forwarding the request to the backend system. Distributed caching is similar to server-side caching, only the response is stored in a distributed cache that is shared across multiple servers.
Share this post
Also, we can use caching so that we don't have to query for data all the time. We just add the version number to the start of the endpoint URL path to version them. If we choose to group users into a few roles, then the roles should have the permissions that cover all they need and no more. If we have more granular permissions for each feature that users have access to, then we have to make sure that admins can add and remove those features from each user accordingly.

case "development":
If we don’t follow commonly accepted conventions, then we confuse the maintainers of the API and the clients that use them since it’s different from what everyone expects. And to document a possible error case we're only throwing a 5XX error at this point. So under "responses" you can see that I've also defined another documentation for that. Documenting endpoints also helps you to understand them better and "forces" you to think of anything you might have forgotten to implement. Those comments that are inside your codebase are also a great documentation for yourself as the API developer, too. You don't have to visit the docs all the time when you want to know the documentation of a specific endpoint.
Caching
Rate limiting helps improve API performance by limiting the number of requests a client can make to an API in a given time period. This prevents clients from overwhelming the API with requests and helps to ensure that the API is responsive for all clients. Authorization is the process of determining what level of access a user or system should have to a particular resource. In API design, authorization is used to ensure that users can only access the resources that they are authorized to access. This is typically done by defining roles and permissions that specify what actions a user is allowed to perform on a particular resource. The Circuit Breaker is a design pattern used to handle errors and faults in distributed systems.
if (data.wishlistProductIds.indexOf($(this).find('.wishlist-toggle').data('product-id')) > -
They provide structured approaches to handle growth in users, data, and functionality efficiently. Focus on user experience, maintain consistency, prioritize security, provide comprehensive documentation, and adapt to change. API aggregation refers to the practice of combining or consolidating multiple APIs (Application Programming Interfaces) into a single interface or endpoint. This aggregated API typically provides access to the functionalities and data of multiple underlying APIs in a unified manner.
Long Running Operations
We can use the same controllers and services in each version globally. One good practice is to add a path segment like v1 or v2 into the URL. Before we write any API-specific code we should be aware of versioning. Like in other applications there will be improvements, new features, and stuff like that. The script makes sure that the development server restarts automatically when we make changes (thanks to nodemon). The whole business logic will be in the Service Layer that exports certain services (methods) which are used by the controller.
RESTful API design patterns provide the architectural blueprint for creating highly scalable and stateless APIs. The technique of partial response enables clients to request only the fields they wish to receive in a response. This reduces the amount of data transferred, increasing API performance. Implementing support for partial responses and range requests can reduce bandwidth and improve response times.
Partner Resources
In this article, we talk a bit about useful and intuitive design patterns in RestFul Webservice API architecture. In general, design patterns are formalized best practices that a programmer can use to solve common problems when designing an application or system. Below are different elements of design patterns for a REST architecture. A document that is easily accessible within your intranet helps everyone understand the design patterns you’ve already adopted.
Our take on microservices
Each value of the enumeration defines which parts of the resource (whichfields) will be returned in the server's response. Exactly what isreturned for each view value is implementation-defined and shouldbe specified in the API documentation. The operation resource must be returned directly as theresponse message and any immediate consequence of the operation should bereflected in the API. For example, when creating a resource,that resource should appear in LIST and GET methods though the resourceshould indicate that it is not ready for use. When the operationis complete, the Operation.response field should contain the message thatwould have been returned directly, if the method was not long running. Microservice API Patterns (MAP) is a volunteer project focused on thedesign and evolution of Microservice APIs addressing endpoint andmessage responsibility, structure, and quality.
How to Tame Your Service APIs: Evolving Airbnb's Architecture - InfoQ.com
How to Tame Your Service APIs: Evolving Airbnb's Architecture.
Posted: Fri, 11 Jun 2021 07:00:00 GMT [source]
ETags can be either strongly or weakly validated, where weakly validated ETagsare prefixed with W/. In this context, strong validation means that tworesources bearing the same ETag have both byte-for-byte identical content andidentical extra fields (ie, Content-Type). This means that strongly validatedETags permit for caching of partial responses to be assembled later. APIs should avoid using other ways of representingranges, such as (index, count), or [first, last].
In a RESTful API world, the API should be developed to ensure they meet the requirements of the desired use cases provided and faced by users, but without exposing the internal business objects. Design for intent is a strategic design pattern that's intended to influence or result in specific and additional user behaviors. You’re ready to create fantastic APIs and better understand the API design architecture, so join the world’s leading API-first companies on Stoplight’s API design management platform. For more on API RESTful web services examples and best practices in API REST building, check out this blog on REST to learn more about how to build a REST API. Perhaps the most common use of an OpenAPI document is to generate API documentation, especially an API reference.
Our book defines APIs as this “A remote API is aset of well-documented network endpoints that allow internal andexternal application components to provide services to each other. Theseservices help achieve domain-specific goals, for instance, fully orpartially automating business processes. They allow clients to activateprovider-side processing logic or support data exchanges and eventnotifications.”. API design is the intentional process of making decisions about how an API exposes data and functionality to its users. It includes defining endpoints, methods, and resources in a standardized specification format. REST API design patterns include resources as collections or items, and the HTTP methods used are GET, POST, PUT, and DELETE.
Why Design Systems Need APIs - Q&A with Louis Chenais, Chief Evangelist at Specify - InfoQ.com
Why Design Systems Need APIs - Q&A with Louis Chenais, Chief Evangelist at Specify.
Posted: Wed, 21 Jul 2021 07:00:00 GMT [source]
Consistency is important in API design because it helps to make APIs more intuitive, predictable, and easy to use and maintain. Consistent resource naming, error responses, and response codes can reduce confusion and errors, while consistent documentation can improve understanding and adoption. Further, API design patterns help to improve the security and scalability of APIs. Developers can use API design patterns to implement authentication and authorization mechanisms that protect APIs from unauthorized access. API design patterns also help to improve the scalability of APIs by allowing developers to implement caching mechanisms that reduce the load on backend systems. Apidog is a notable tool in the field of API (Application Programming Interface) design, playing a key role in guiding developers through the nuances of creating efficient, robust, and user-friendly APIs.
However, you can go even further by enforcing your style guide programmatically. Using a tool like an open-source linter, you can define rulesets for your OpenAPI documents. These patterns provide a set of pre-established solutions to common API development problems, helping developers to build robust APIs faster. Think of them as a blueprint or a set of reusable solutions that can be applied to solve common problems encountered when designing APIs. Just like software, design pattern refers to a particular system structure that can be reused to solve commonly occurring software problems. Application Programming Interfaces (APIs) allow different applications to communicate with each other and share data, enabling developers to create complex systems that work together seamlessly.
The reason for this recommendation is because clients oftenreuse resources returned by the server as another request input, e.g. aretrieved Book will be later reused in an UPDATE method. If output only fieldsare validated against, then this places extra work on the client to clear outoutput only fields. Each pattern text then can be seen as a small specialized article inits own,3 usually a few pages long andconsisting of 2000 to 3000 words .4 These texts arestructured according to a standard template. For instance, the templatesuggests to direct readers to the next patterns that become eligible andinteresting once a particular one has been studied and applied. Forinstance,EmbeddedEntity suggests to addLinkedInformation Holders when relationships have to be followed on theclient side, but the messages get too big if all referenced data isincluded. Pattern languages such as Enterprise Integration Patterns and CloudComputing Patterns provide such “next” pointers too.
The first and absolute must have is to use SSL/TLS because it's a standard nowadays for communications on the internet. It's even more important for API's where private data is send between the client and our API. We've spoken about best practices to increase the usability and performance of our API. You can build the best API, but when it is a vulnerable piece of software running on a server it becomes useless and dangerous. Inside there you can define how long your data should be cached. The time depends on how fast or how often your data inside your cache changes.
No comments:
Post a Comment